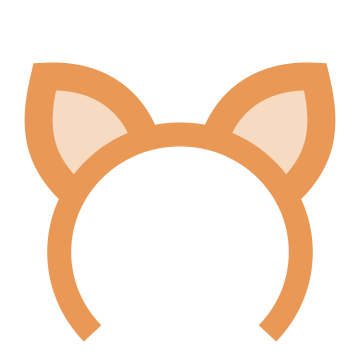
maomi
Strict and Performant Web Application Programming
#[component]
struct HelloWorld {
template: template! {
"Hello world!"
}
}
Programming in Rust
Write rust code, compile to WebAssembly, and run in browser.
// declare a component
#[component(Backend = DomBackend)]
struct HelloWorld {
// write a template
template: template! {
<div class:hello>
"Hello world!"
</div>
}
}
// implement Component trait
impl Component for HelloWorld {
fn new() -> Self {
Self {
template: Default::default()
}
}
}
// write styles
stylesheet! {
class hello {
color = rgb(232, 152, 86);
}
}
Better Performance
Maomi has great overall performance and avoids common performance pitfalls. Like rust language itself, there is no worry about performance in maomi application programming.
tree build timing
tree update timing
component abstraction timing
Report Mistakes while Compilation
Like rust, maomi reports mistakes while compilation. The mistakes include wrong element names, invalid properties, and even wrong style class names.
Work with rust-analyzer in IDE
With rust-analyzer installed, it is much easier to investigate elements, properties, and even style classes.
Data Bindings
Maomi is based on templates and data bindings.
#[component]
struct HelloWorld {
template: template! {
<div class:hello>
// use struct fields in the template
{ &self.hello }
</div>
},
hello: String,
}
Limited CSS
Maomi supports a limited stylesheet syntax. It restricts the usage of CSS to make the styling easier to investigate.
stylesheet! {
class hello {
color = rgb(232, 152, 86);
if hover {
text_decoration = underline;
}
}
}
High Performance Server Side Rendering
Maomi supports server side rendering. It just execute native rust binary in the server to generate HTML output. It is much more performant than using a JavaScript runtime.
Integrated i18n Support
Maomi supports i18n in the core design. It is easy to compile with TOML-based translation files to generate different version of the application.